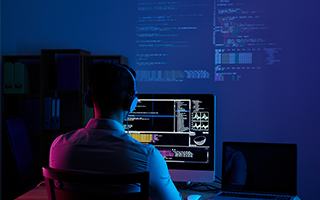
Full Stack Development
Unlock the power of Full Stack Development with hands-on projects and real-world applications, enhancing problem-solving skills and paving the way for innovative solutions.
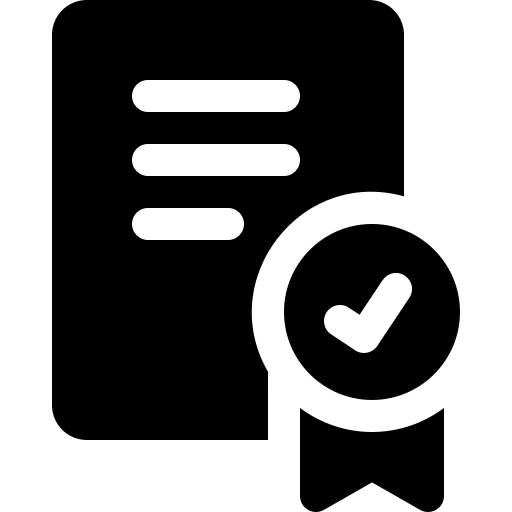
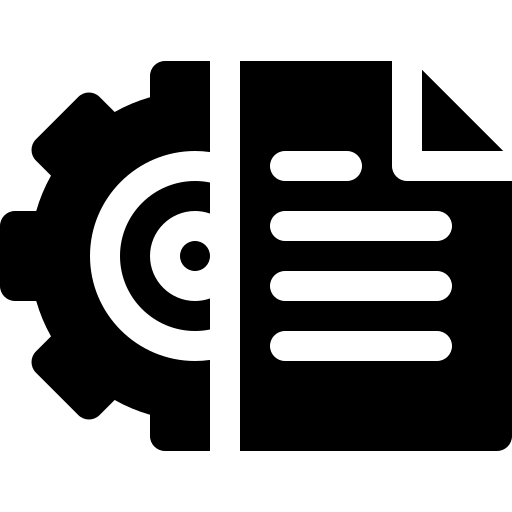
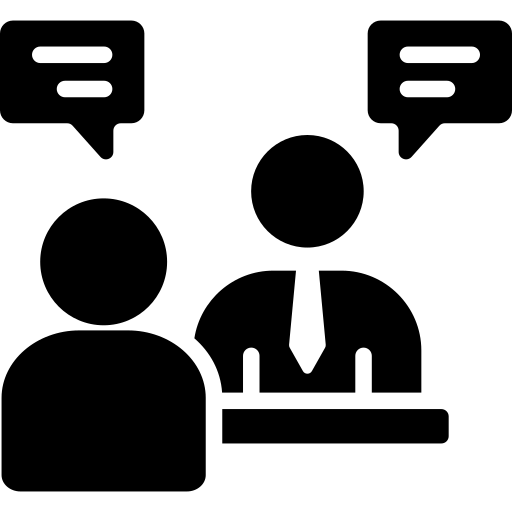
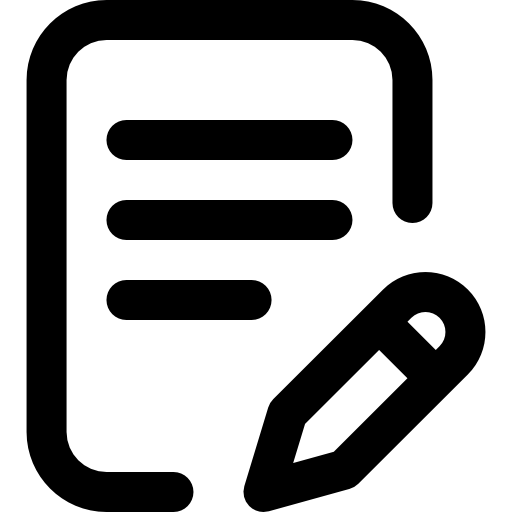





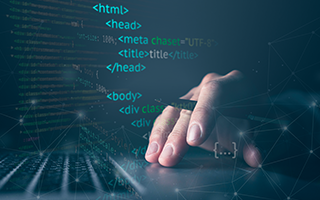
Web Development
Unlock your web development potential by mastering React, build dynamic websites, and launch your tech career today.
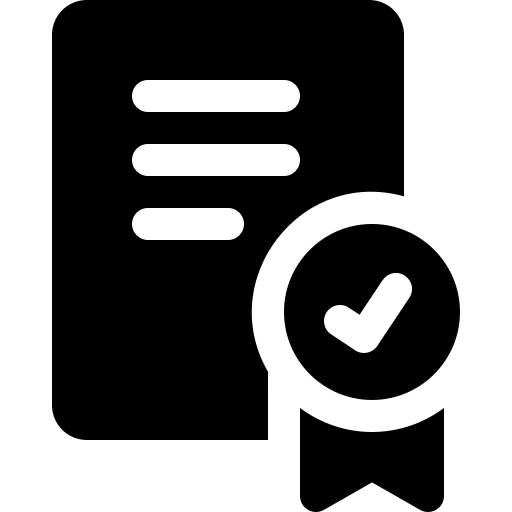
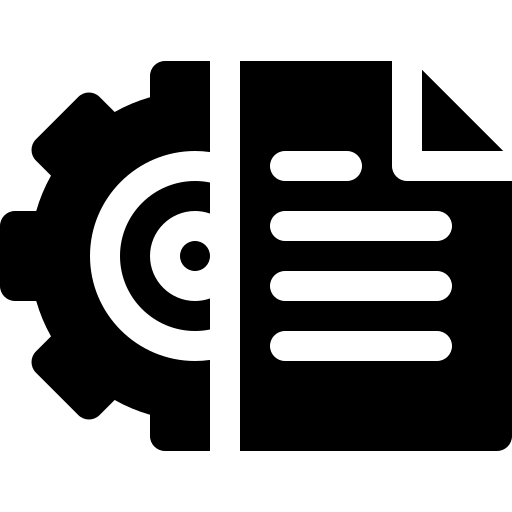
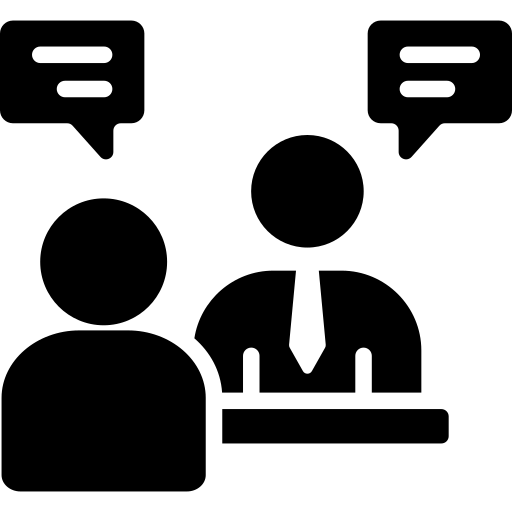
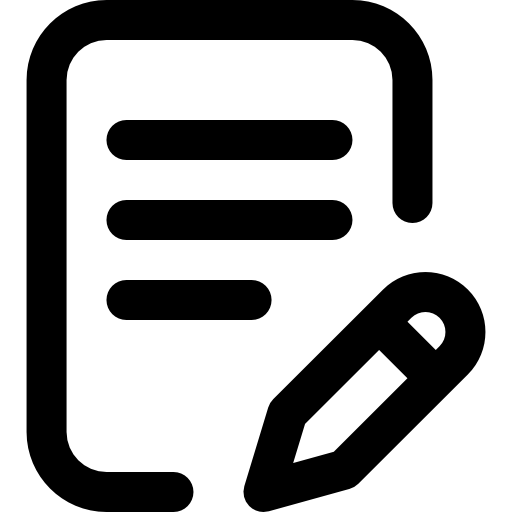





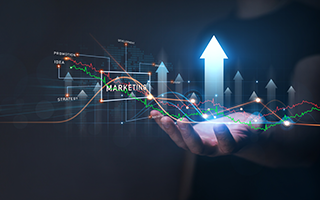
Digital Marketing
Get ready for marketing in the digital age & strategize for the Modern Businesses.
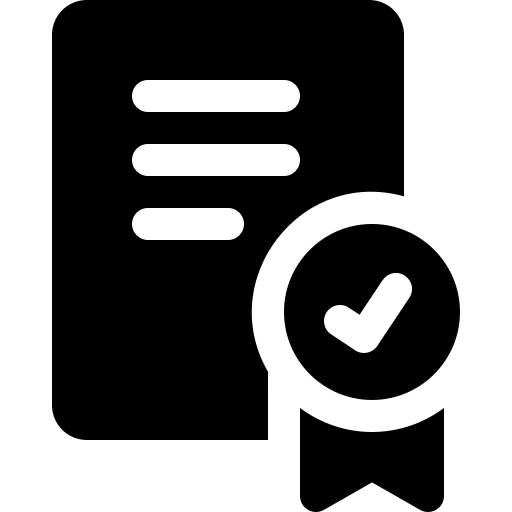
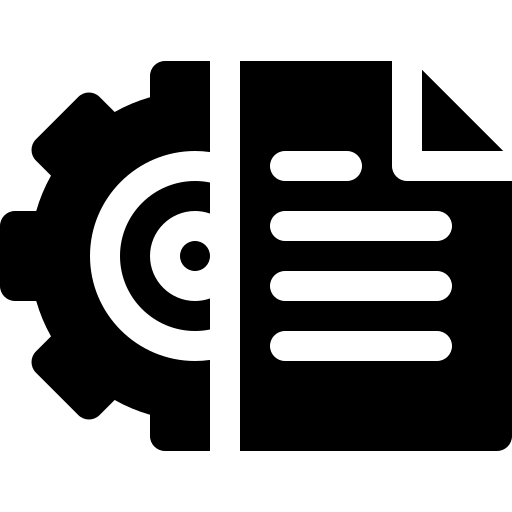
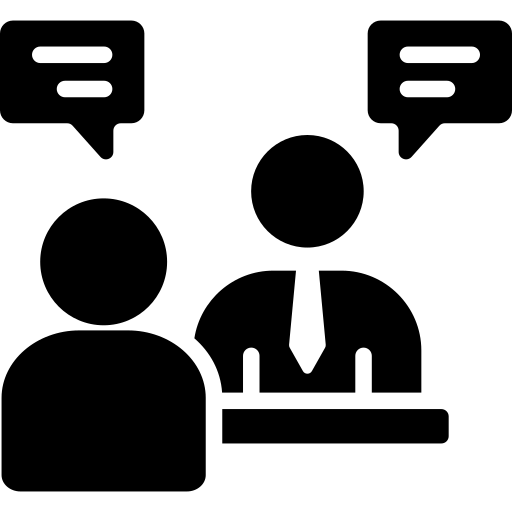
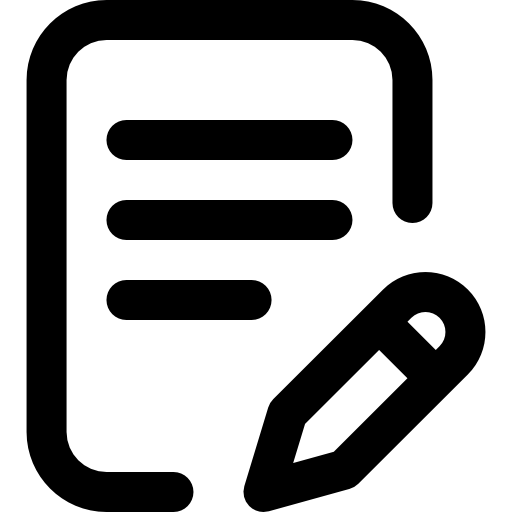





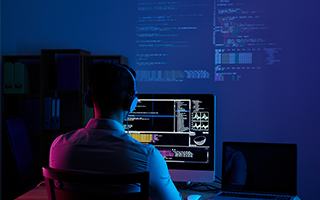
Python
Explore Python language's versatility and learn to code efficiently
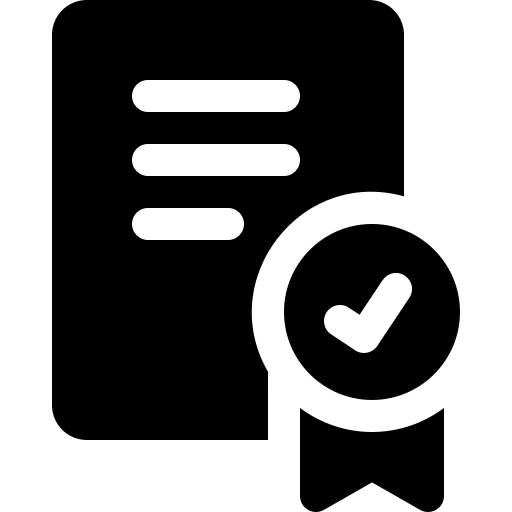
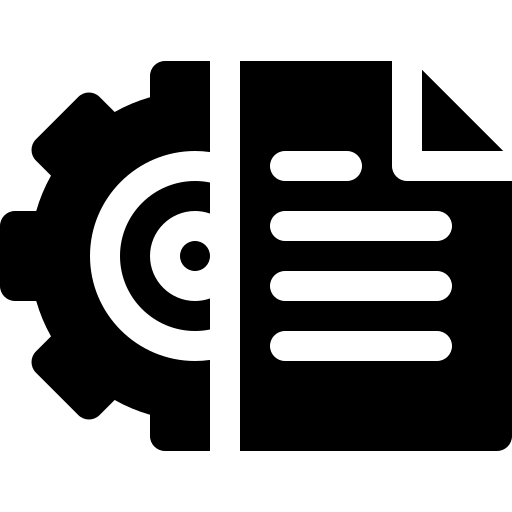
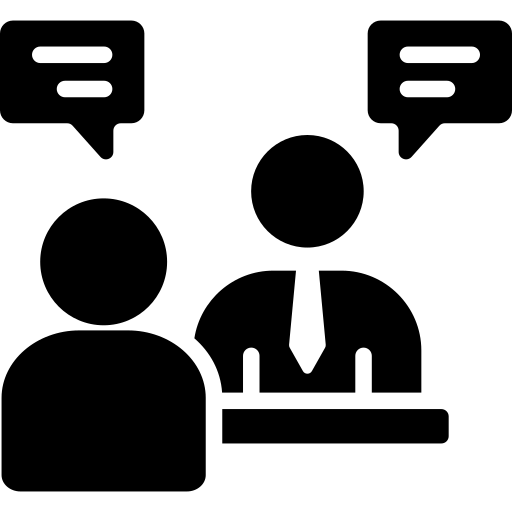
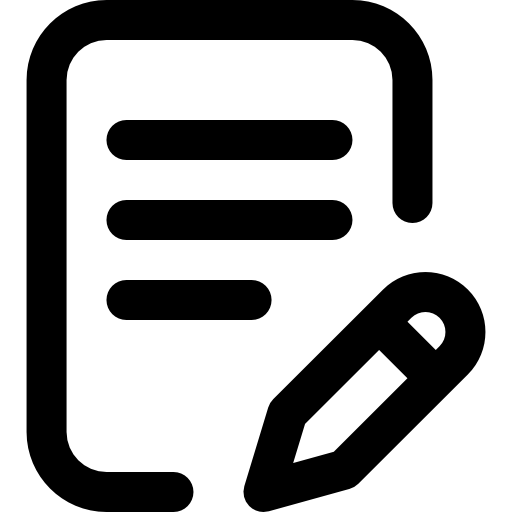





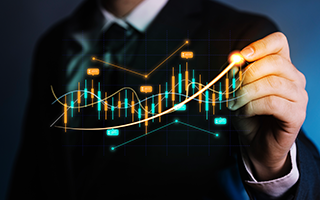
Finance
Understand the ways to strategize for the financial fitness of the organization.
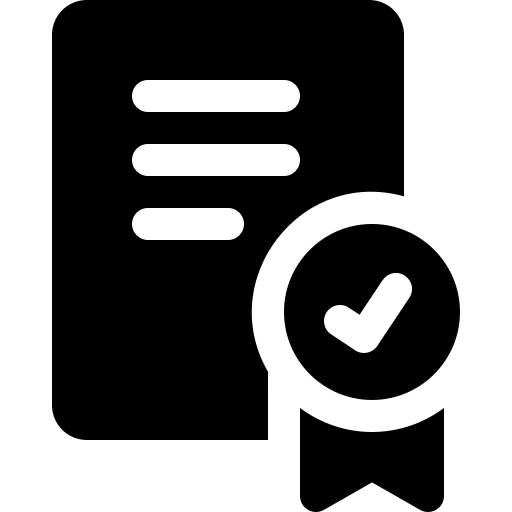
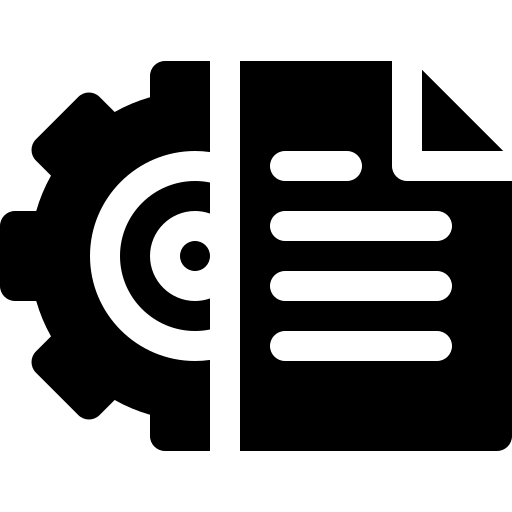
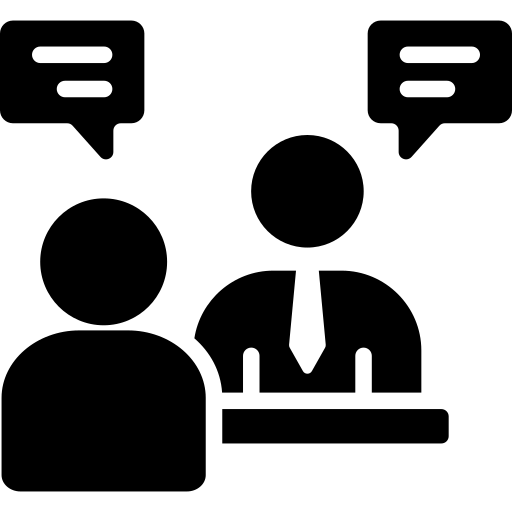
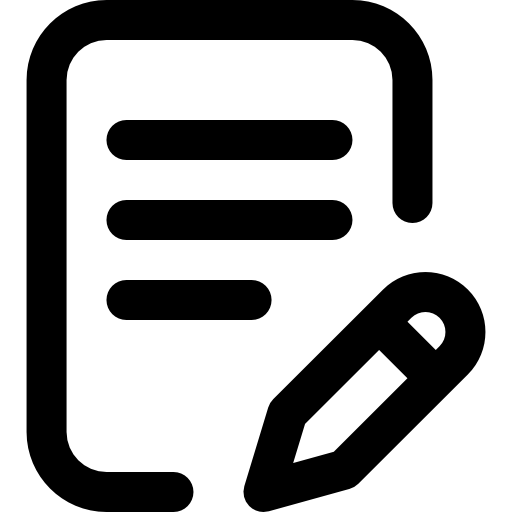





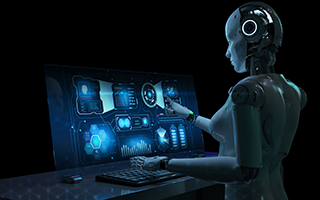
Machine Learning
Harness the power of algorithms, unlock data insights, and shape the innovations with Machine Learning Program.
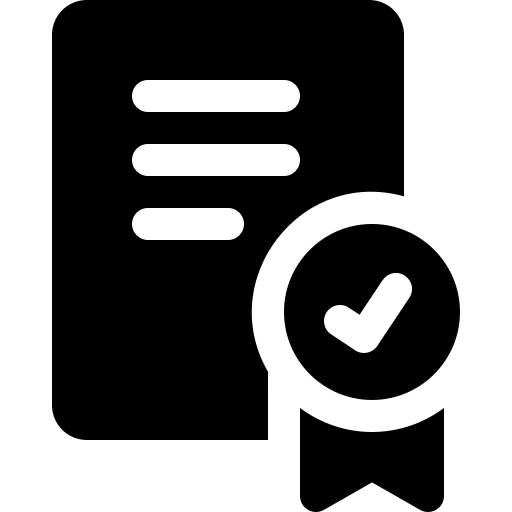
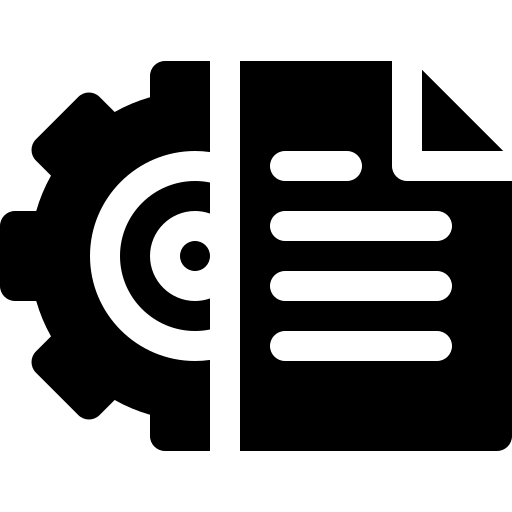
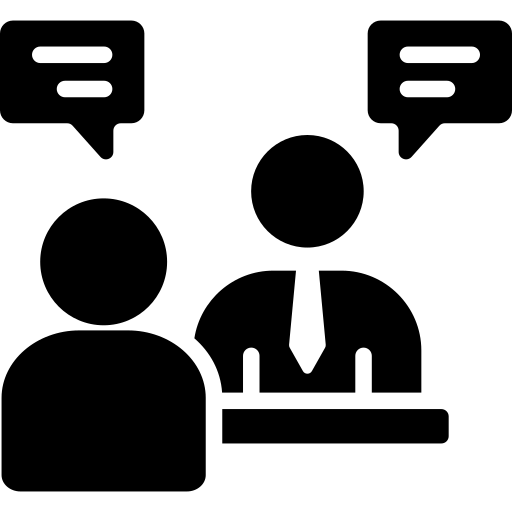
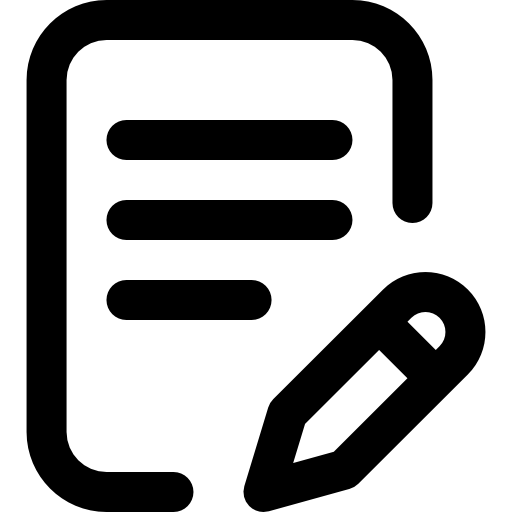





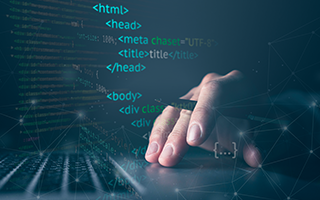
Web Development
Unlock your web development potential by mastering React, build dynamic websites, and launch your tech career today.
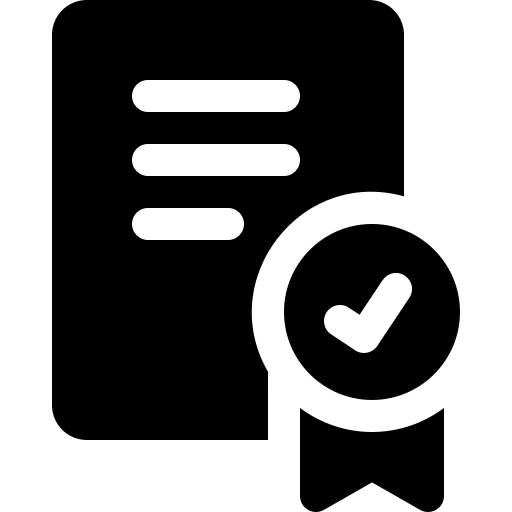
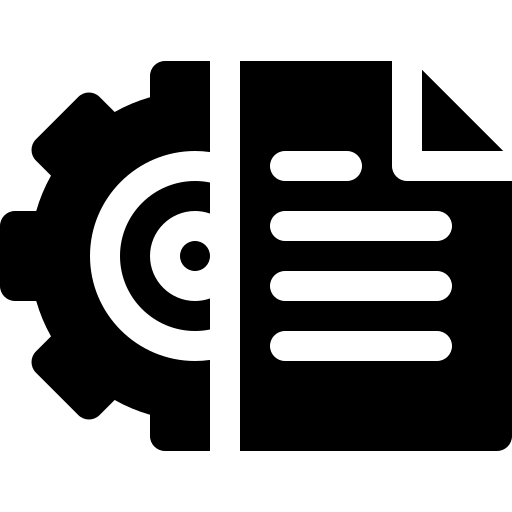
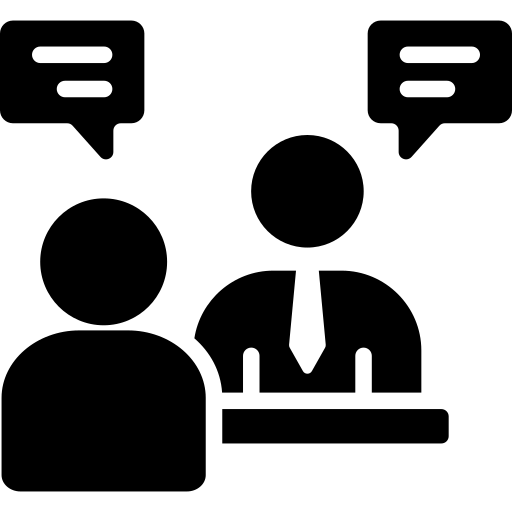
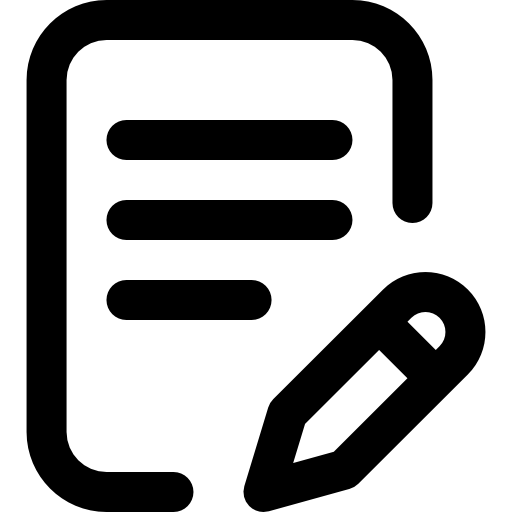





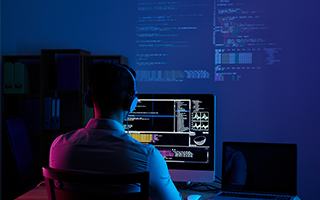
E-commerce Developer Bootcamp
Learn to design, develop, and deploy high-performing E-commerce websites that captivate customers and increase conversions.





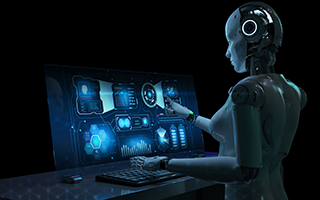
Machine Learning
Harness the power of algorithms, unlock data insights, and shape the innovations with Machine Learning Program.
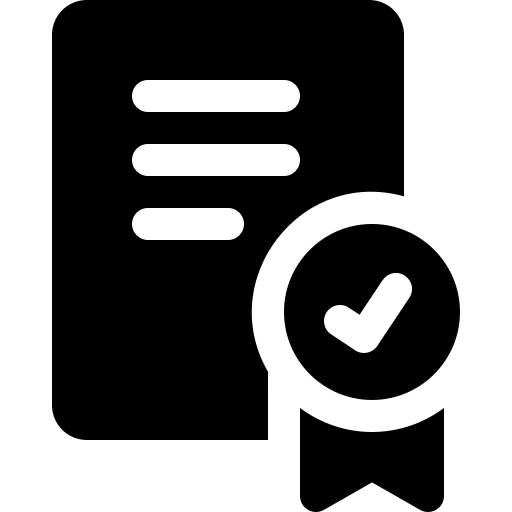
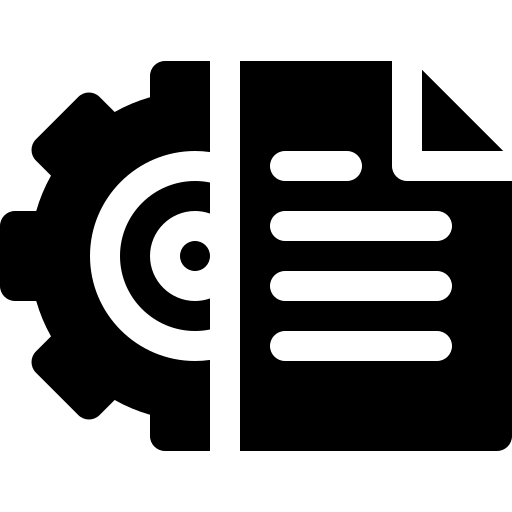
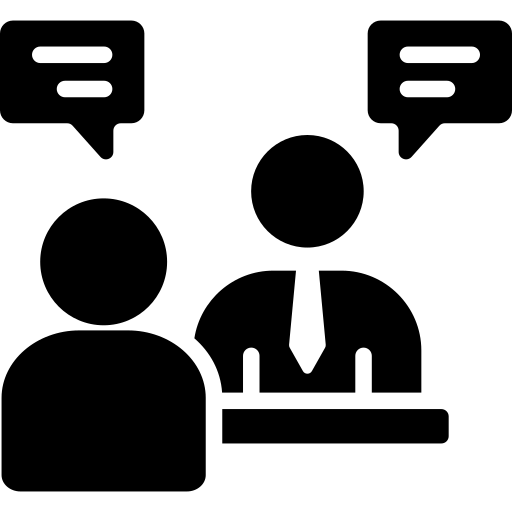
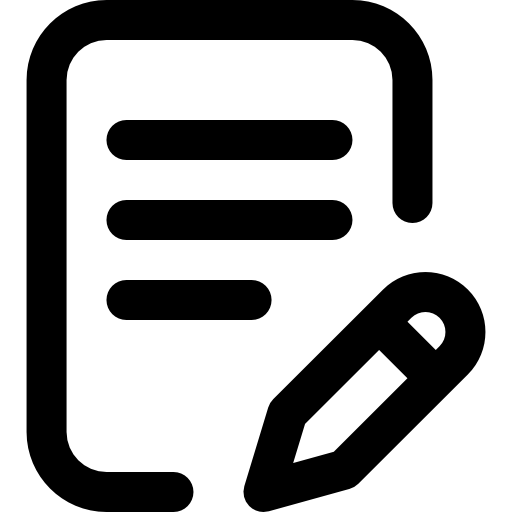





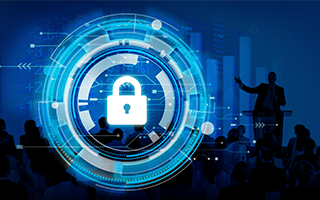
Cyber Security
Build a strong foundation for Digital Safety by mastering Cyber Security
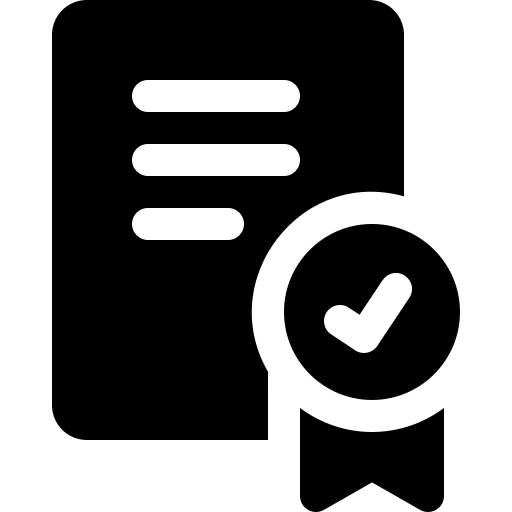
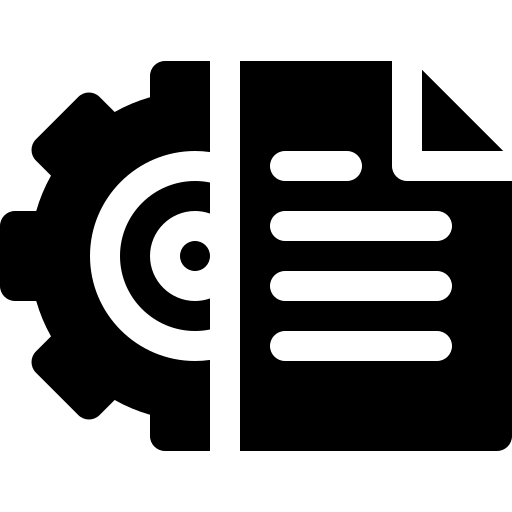
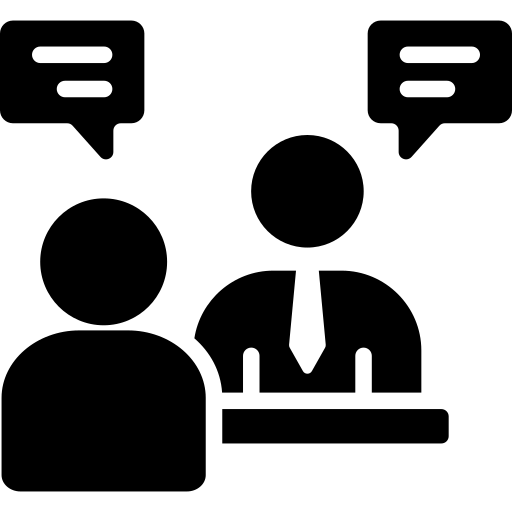
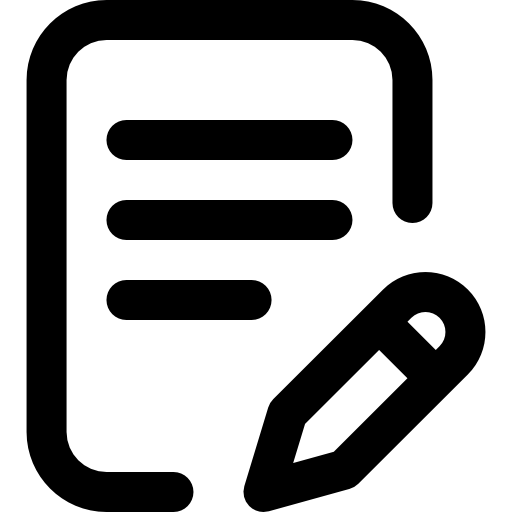





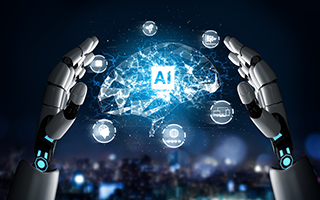
Artificial Intelligence
Master the fundamentals and cutting-edge applications through our comprehensive course designed for aspiring AI enthusiasts.
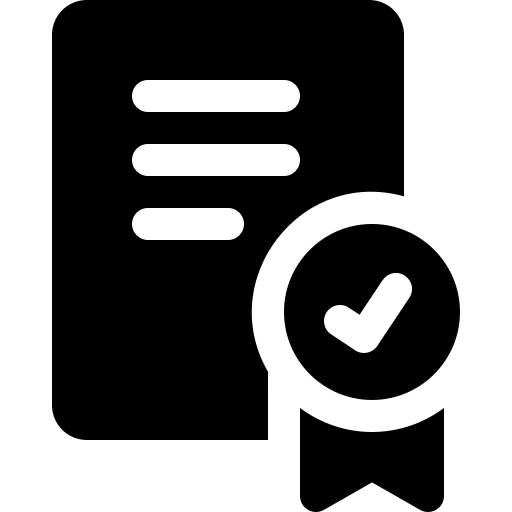
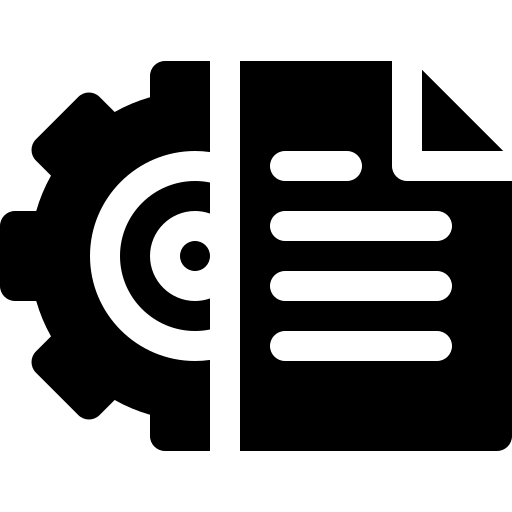
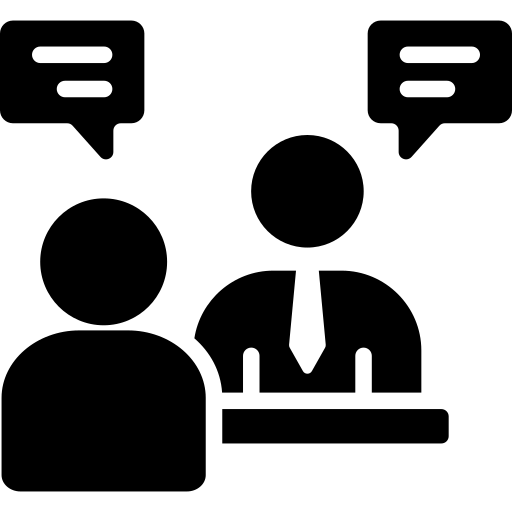
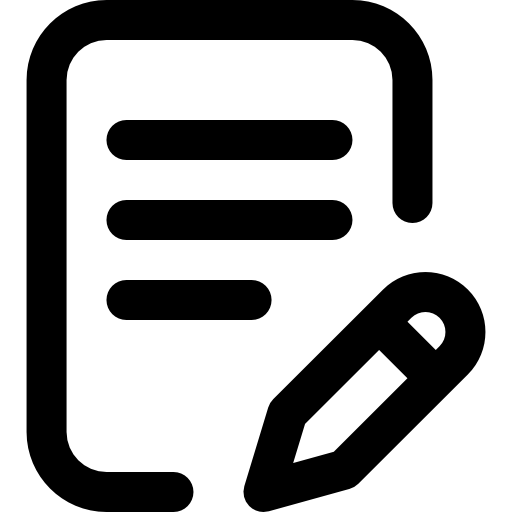





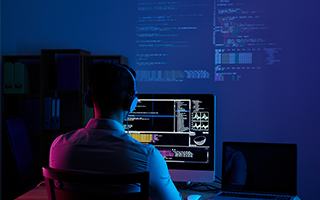
Python
Explore Python language's versatility and learn to code efficiently
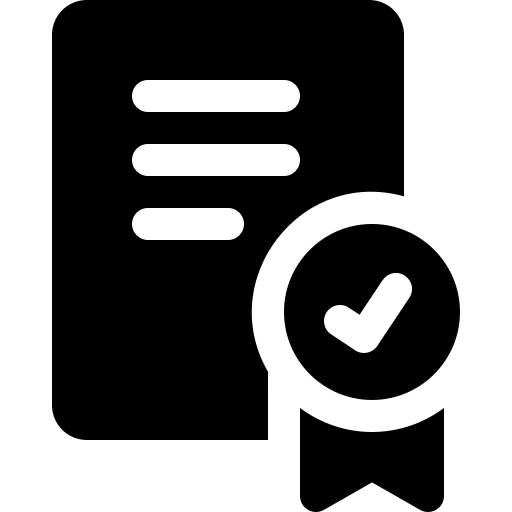
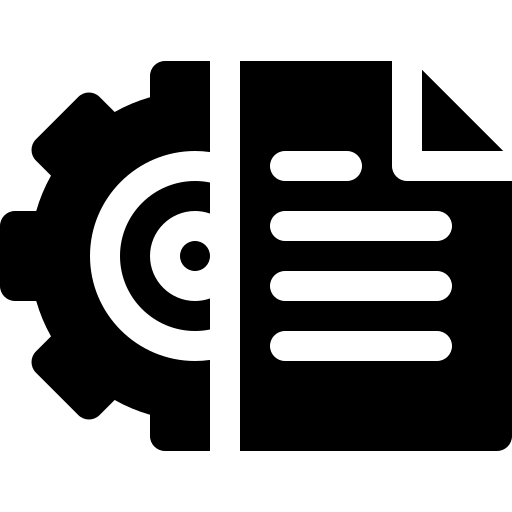
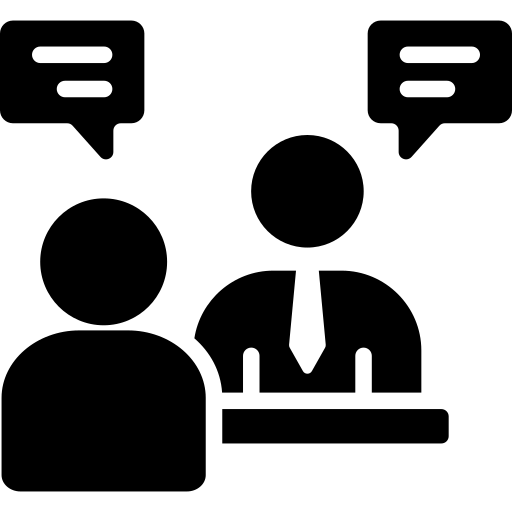
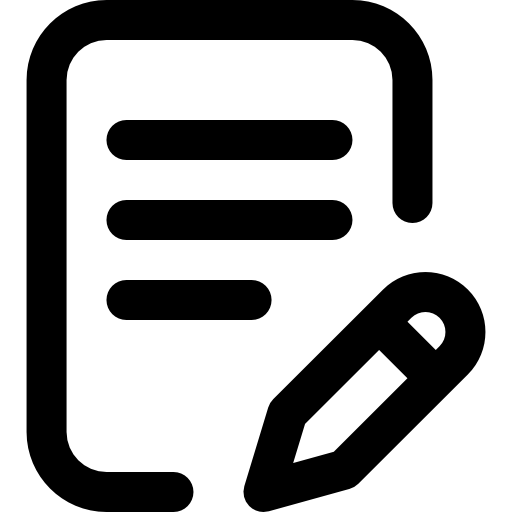





View More
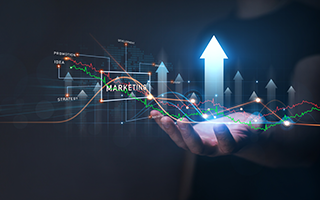
Digital Marketing
Get ready for marketing in the digital age & strategize for the Modern Businesses.
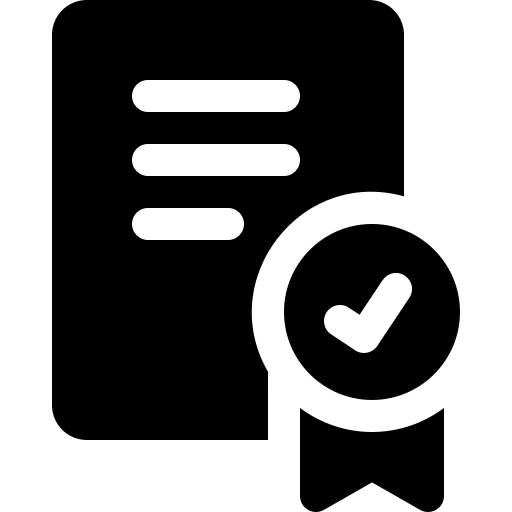
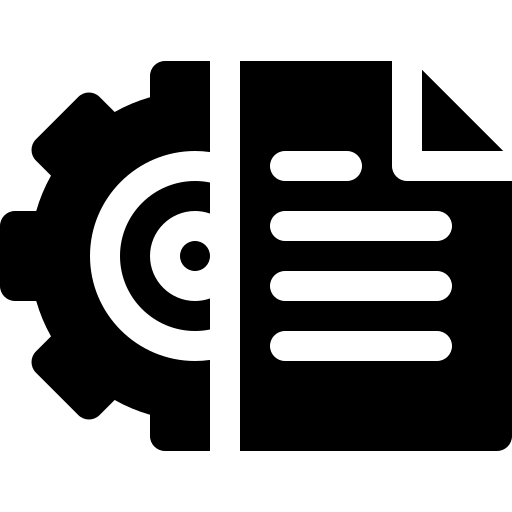
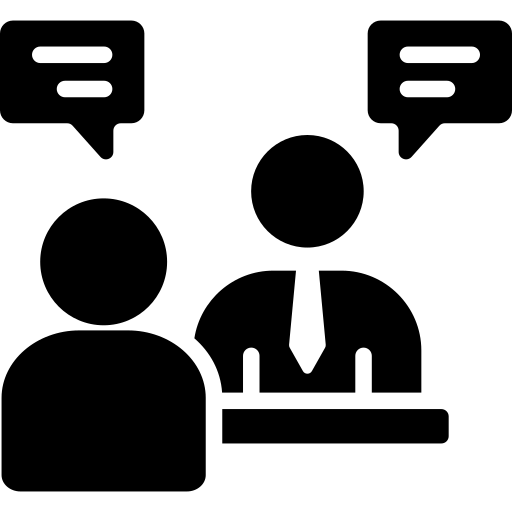
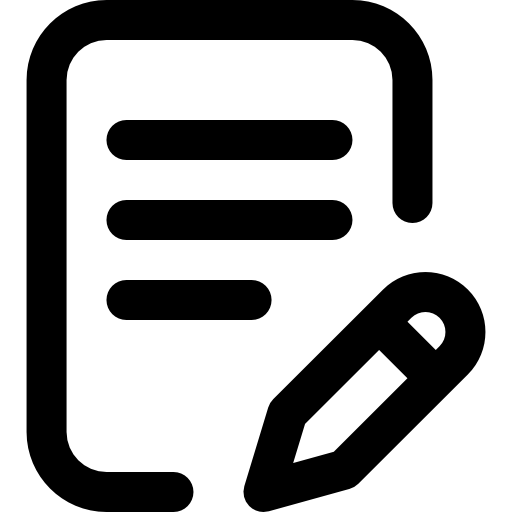





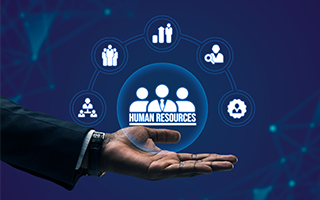
HR
Learn the science of human resources management to elevate the efficiency of the organization.
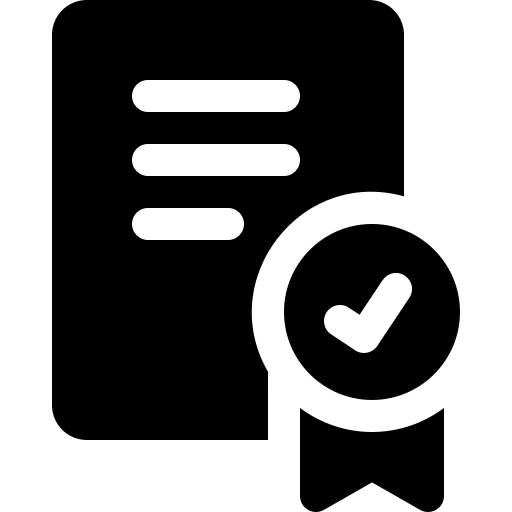
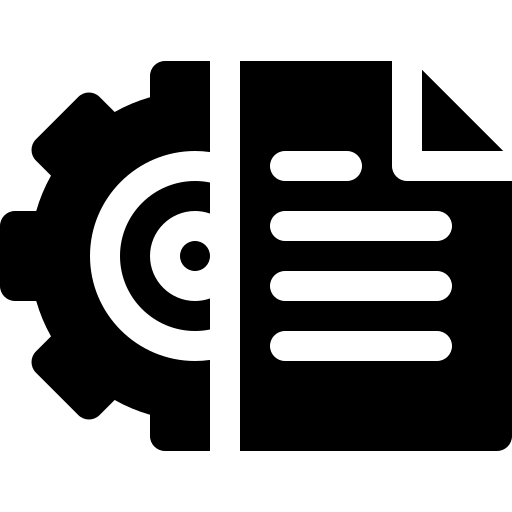
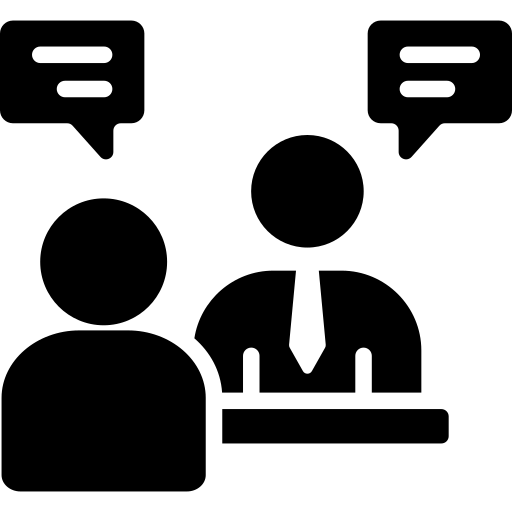
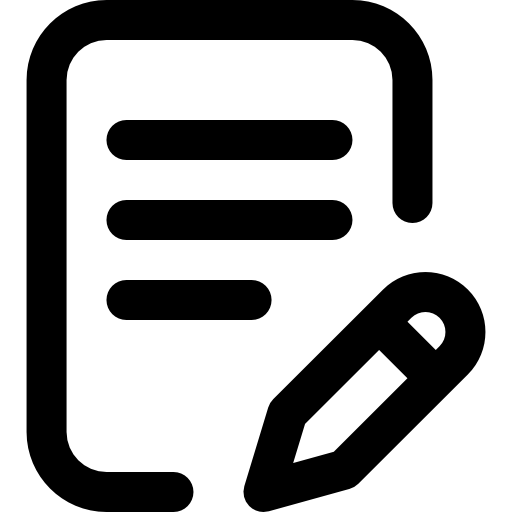





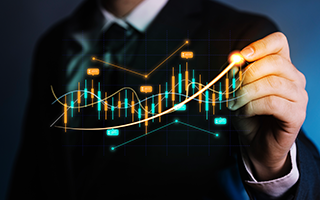
Finance
Understand the ways to strategize for the financial fitness of the organization.
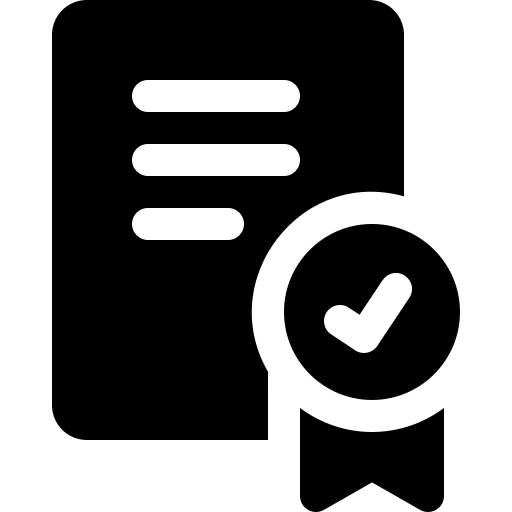
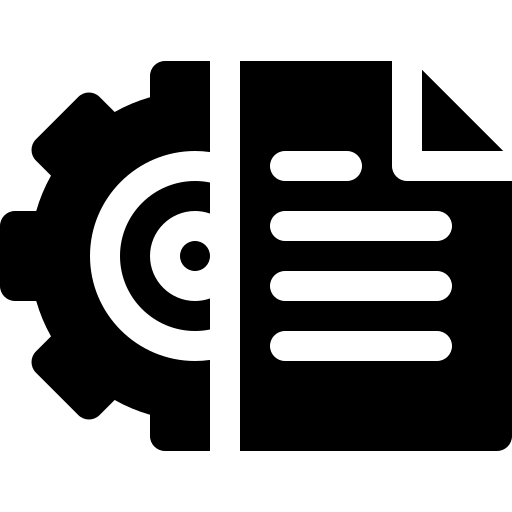
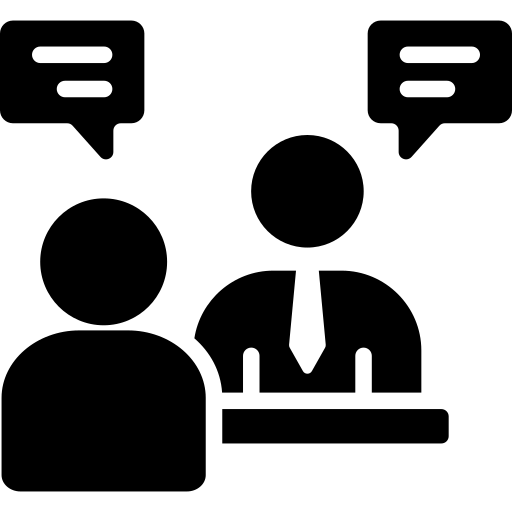
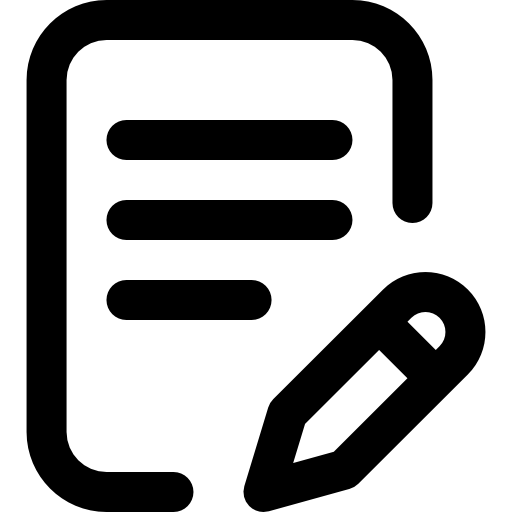





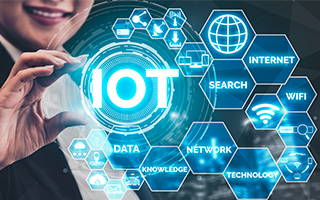
IoT & Robotics
Learn the science behind the integration of the physical & virtual world through IoT and Robotics.
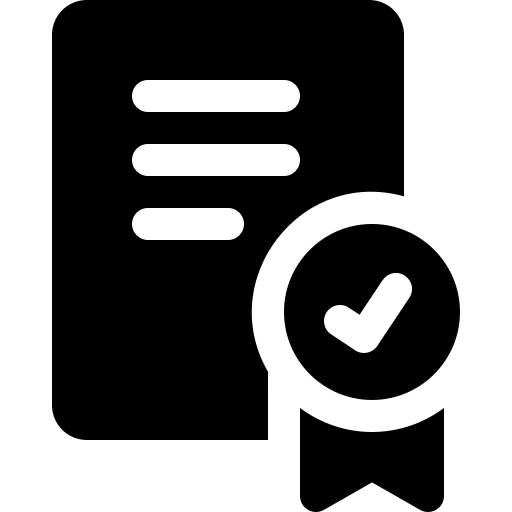
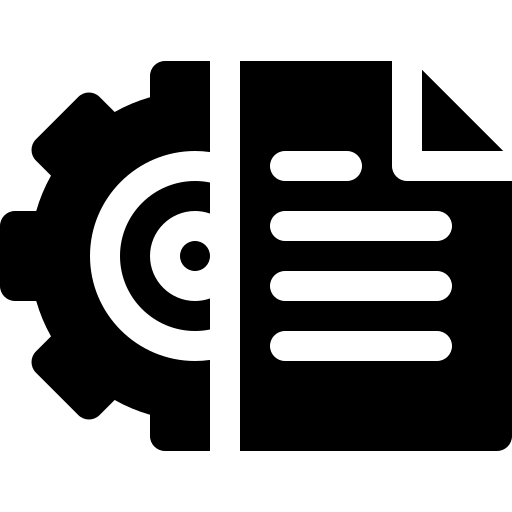
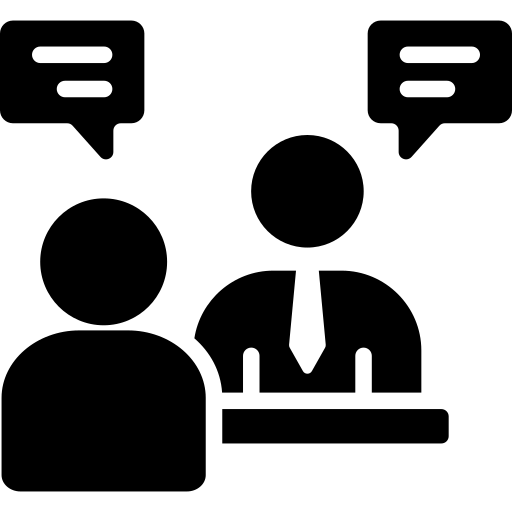
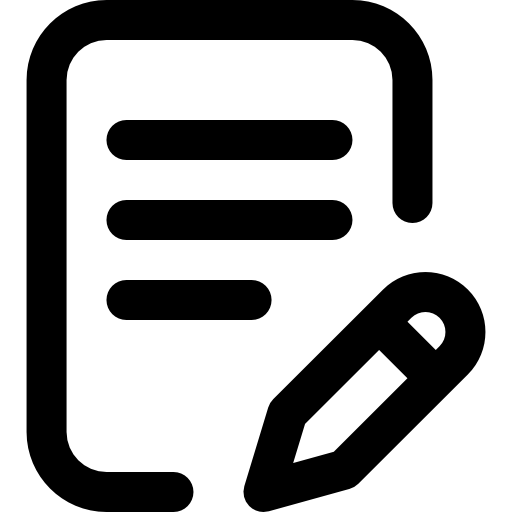





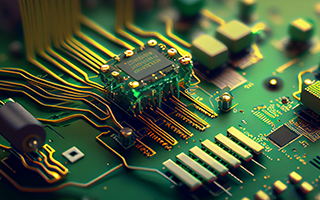
Embedded System
Gain the knowledge to design & build smart devices for tomorrow.
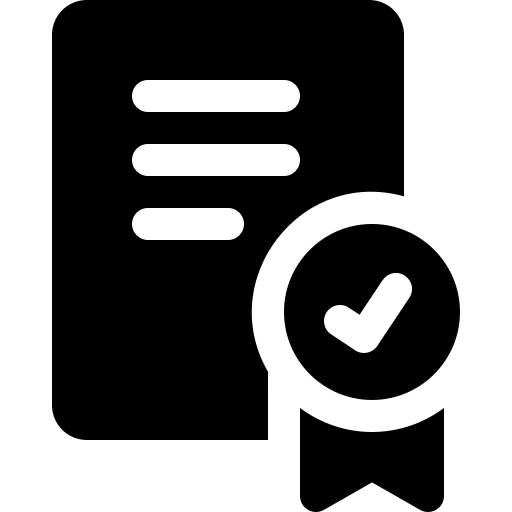
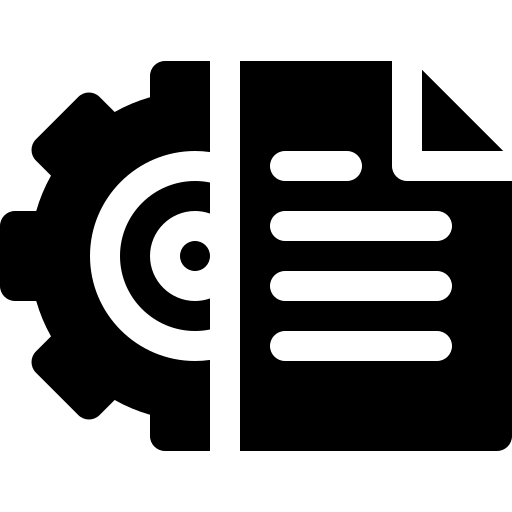
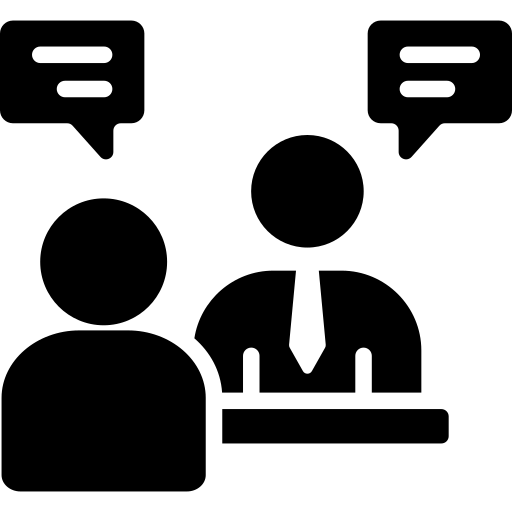
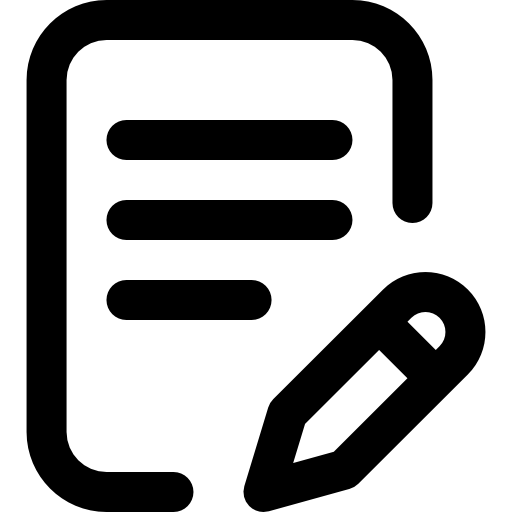




