Table of Contents
Taking input is a method that is used to interact with the user to get some result. This article is about how to take input in Python.
Every computer application needs to accept input from the user when it is running. Further, this will make the application more interactive. This majorly will depend on how it is developed, an application will accept the user input in the form of text entered in the console (sys. stdin), a graphical layout, or a web-based interface.
Python user input
Python has two built-in methods to read the data from keywords. Those methods include
a. raw(prompt)
b. raw_input
input()
This input function uses the latest version of Python. Further, it will take input from the user and check the expression. Whereas, the Python interpreter will analyze whether the user inputs a string, number, or list.
Example
# Python program showing
# a use of input()
name = input("Enter your name: ")
print(name)
Output
Enter your name: Albert
Devansh
Here, the Python interpreter won’t run the further line until the user enters the input.
Example 2
# Python program showing
# a use of input()
name = input("Enter your name: ") # String Input
age = int(input("Enter your age: ")) # Integer Input
marks = float(input("Enter your marks: ")) # Float Input
print("The name is:", name)
print("The age is:", age)
print("The marks is:", marks)
Output
Enter your name: Albert
Enter your age: 22
Enter your marks: 89
The name is: Albert
The age is 22
The marks is: 89.0
This example will tell us that the input function will take input as a string and then it is required to enter the integer.
How input function works?
The flow of the program will be put to an end until the user enters the input. The text statement is referred to as a prompt and is not mandatory to write the input function. So, the prompt will show the message on the console.
However, the input () function will turn the user input into a string. It is necessary to turn the input with the help of typecasting. The raw input function will be used in the Python’s older version such as Python 2,x. It will take the input from the keyword and will be returned as a string.
Example
# Python program showing
# a use of raw_input()
name = raw_input("Enter your name : ")
print name
Output
Enter your name: John
John
How to check the Python version?
It is required to open the command lines in Windows, shell, or Mac to check the Python version. Then, we need to execute the Python version. So, it will be shown in the corresponding Python version.
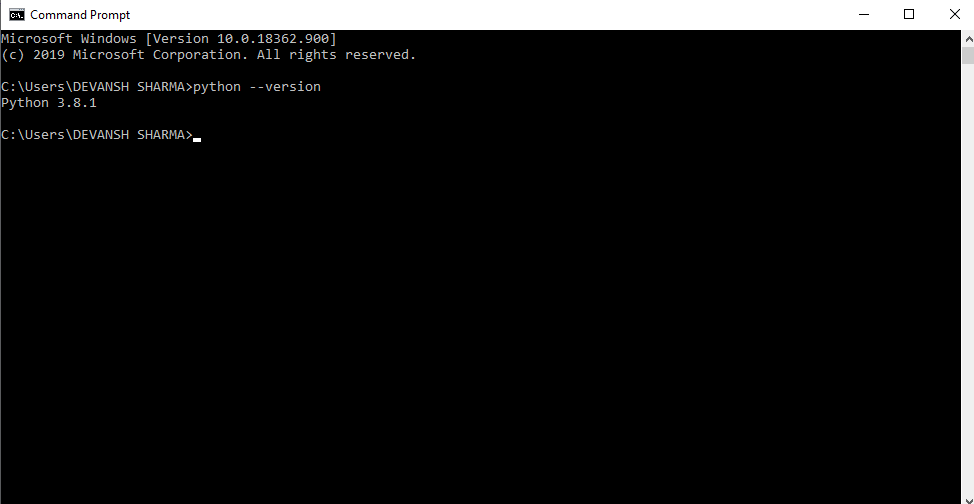
How to check the Python version in the running Script?
The following methods are used to check the Python version in every operating system
hon –version or Python -v or Python -vv | Window/Mac/Linux | Python 3.8.1 |
import sys sys.version sys.version_info | Python Script | 3.8.3 (default, May 13 2020, 15:29:51) [MSC v.1915 64 bit (AMD64)] |
Import platform platform.python_version() | Python Script | ‘3.8.1’ |
Conclusion
To conclude, this article will allow us to improve our skills and knowledge about Python user input. It has also included ways to check the Python version.
How to take input in Python- FAQs
Q1. What does input () mean in Python?
Ans. It refers to allowing a user to provide a value to the program.
Q2. Is input () in Python a string?
Ans. In Python, whatever you had provided as an input. Then, the input function will be turned into a string.
Q3. How to use int in Python?
Ans. int mainly functions in the int() built-in function.
Hello, I’m Hridhya Manoj. I’m passionate about technology and its ever-evolving landscape. With a deep love for writing and a curious mind, I enjoy translating complex concepts into understandable, engaging content. Let’s explore the world of tech together